R Package
This article details how to install, and use the R-package with the ZENTRA Cloud API.
You can use your API Token, device serial number, and start and end date to query data.
Installation
The package is hosted on a public Gitlab page. To install the package:
url = "https://gitlab.com/meter-group-inc/pubpackages/zentracloud"
remotes::install_git(url = url)
Server
US
setZentracloudOptions(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, domain = "default"
)
EU
setZentracloudOptions(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, domain = "EU"
)
CN
setZentracloudOptions(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, domain = "CN"
)
TAHMO
setZentracloudOptions(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, domain = "tahmo"
)
Examples
User-relevant, exported functions:
functions for token management
setZentracloudToken
writes a token to the options. Afterward, the token can easily be retrieved and used in further functions. This function has to be performed once at the beginning of a session, but can also be written into the .Rprofile.
setZentracloudToken(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, overwrite = TRUE
)
token = getZentracloudToken()
Token format
Sys.setenv(ZENTRACLOUD_TOKEN = "757575e7caca75e75b0cd7575fea75b757575")
functions to get data
getReadings
is the main function of the package. It takes a start and end date, as well as the serial number of the device in question, and a valid token.
It first checks the cache to see if the requested time period is covered by the results of a previous query. If yes, it loads that portion from the cache and fetches the new data, while also writing the new data to the cache. If the time period is not covered in the previous results, then it queries the ZENTRA Cloud API and saves the results to the cache.
library(zentracloud)
setZentracloudToken(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, overwrite = TRUE
)
zentra_data = getReadings(
device_sn = "06-01185"
, token = getZentracloudToken()
, start_time = "2022-06-01 00:00:00"
, end_time = "2022-06-14 23:59:00"
, cache = "default"
, domain = "default"
, force = FALSE
)
str(zentra_data, max.level = 2)
#> List of 6
#> $ ATM-410003090_port6:'data.frame': 20 obs. of 18 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Air Temperature : num [1:20] 12.1 11.6 11.6 11.5 11.5 11.5 11.2 11.2 11.1 11.5 ...
#> ..$ Atmospheric Pressure: num [1:20] 93 93 93 93 93 ...
#> ..$ Gust Speed : num [1:20] 5 2.96 3.34 4 4.08 4.75 2.75 3.14 3.96 5.45 ...
#> ..$ Lightning Activity : num [1:20] 0 0 0 0 0 0 0 0 0 0 ...
#> ..$ Lightning Distance : num [1:20] 0 0 0 0 0 0 0 0 0 0 ...
#> ..$ Max Precip Rate : num [1:20] 0 0 0 0 0 0 0 0 0 0 ...
#> ..$ Precipitation : num [1:20] 0 0 0 0 0 0 0 0 0 0 ...
#> ..$ RH Sensor Temp : num [1:20] 12 11.8 11.4 11.3 11.2 11.2 11.2 11 10.9 10.9 ...
#> ..$ Solar Radiation : num [1:20] 0 0 0 0 0 0 0 0 0 0 ...
#> ..$ VPD : num [1:20] 0.39 0.35 0.36 0.35 0.35 0.35 0.31 0.31 0.3 0.34 ...
#> ..$ Vapor Pressure : num [1:20] 1.02 1.02 1 1.01 1.01 ...
#> ..$ Wind Direction : num [1:20] 105 114 107 106 98 99 101 102 95 96 ...
#> ..$ Wind Speed : num [1:20] 2.93 1.86 2.03 2.24 2.08 2.27 1.63 1.61 1.69 2.63 ...
#> ..$ X-axis Level : num [1:20] -0.6 -0.7 -0.7 -0.7 -0.7 -0.7 -0.7 -0.6 -0.7 -0.6 ...
#> ..$ Y-axis Level : num [1:20] 0.8 0.8 0.8 0.8 0.8 0.9 0.9 0.8 0.8 0.8 ...
#> ..- attr(*, "sensor")= chr "ATM-410003090_port6"
#> $ T12-0000248_port5 :'data.frame': 20 obs. of 6 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Saturation Extract EC: num [1:20] 2.1 2.09 2.1 2.1 2.1 ...
#> ..$ Soil Temperature : num [1:20] 10.1 10.1 10.1 10.1 10.1 10.1 10.1 10.1 10.1 10.1 ...
#> ..$ Water Content : num [1:20] 0.361 0.361 0.361 0.361 0.361 0.361 0.361 0.361 0.361 0.361 ...
#> ..- attr(*, "sensor")= chr "T12-0000248_port5"
#> $ T12-0000495_port4 :'data.frame': 20 obs. of 6 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Saturation Extract EC: num [1:20] 1.86 1.86 1.86 1.86 1.86 ...
#> ..$ Soil Temperature : num [1:20] 10.7 10.7 10.7 10.7 10.7 10.7 10.7 10.7 10.7 10.7 ...
#> ..$ Water Content : num [1:20] 0.348 0.348 0.348 0.348 0.348 0.348 0.348 0.348 0.348 0.348 ...
#> ..- attr(*, "sensor")= chr "T12-0000495_port4"
#> $ T12-0000499_port2 :'data.frame': 20 obs. of 6 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Saturation Extract EC: num [1:20] 1.47 1.47 1.47 1.47 1.47 ...
#> ..$ Soil Temperature : num [1:20] 12.2 12.2 12.2 12.2 12.2 12.2 12.3 12.3 12.3 12.3 ...
#> ..$ Water Content : num [1:20] 0.334 0.334 0.334 0.334 0.334 0.334 0.334 0.334 0.334 0.334 ...
#> ..- attr(*, "sensor")= chr "T12-0000499_port2"
#> $ T12-0000500_port1 :'data.frame': 20 obs. of 6 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Saturation Extract EC: num [1:20] 0.977 0.977 0.977 0.972 0.969 0.968 0.97 0.971 0.971 0.976 ...
#> ..$ Soil Temperature : num [1:20] 13.8 13.8 13.8 13.8 13.8 13.8 13.8 13.8 13.7 13.7 ...
#> ..$ Water Content : num [1:20] 0.291 0.291 0.291 0.291 0.291 0.291 0.291 0.291 0.291 0.291 ...
#> ..- attr(*, "sensor")= chr "T12-0000500_port1"
#> $ T12-0000504_port3 :'data.frame': 20 obs. of 6 variables:
#> ..$ timestamp_utc : int [1:20] 1654066800 1654067700 1654068600 1654069500 1654070400 1654071300 1654072200 1654073100 1654074000 1654074900 ...
#> ..$ datetime : POSIXct[1:20], format: "2022-06-01 00:00:00" "2022-06-01 00:15:00" ...
#> ..$ tz_offset : int [1:20] -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 -25200 ...
#> ..$ Saturation Extract EC: num [1:20] 1.18 1.19 1.19 1.19 1.19 ...
#> ..$ Soil Temperature : num [1:20] 11.5 11.5 11.5 11.5 11.5 11.5 11.6 11.6 11.6 11.6 ...
#> ..$ Water Content : num [1:20] 0.328 0.328 0.328 0.328 0.328 0.328 0.328 0.328 0.328 0.328 ...
#> ..- attr(*, "sensor")= chr "T12-0000504_port3"
functions to clear the cache
clearCache
allows clearing the cache of files older than the specified file_age.
# deletes all .parquet files that are 30 days or more old
clearCache(file_age = 30L)
functions to get the settings
queryDeviceSettings
will query the API and return the contents of the respective endpoint in JSON:
library(listviewer)
settings = queryDeviceSettings(
token = getZentracloudToken()
, domain = "default"
, device_sn = "06-01185"
)
jsonedit(settings)
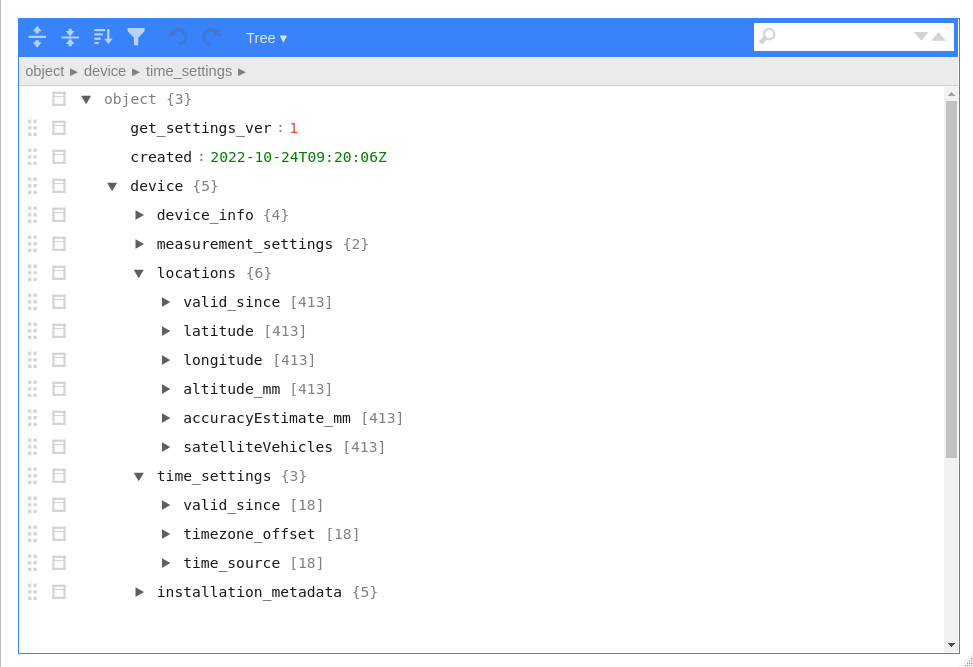
Benchmarks for cache vs. API
setZentracloudToken(
token = Sys.getenv("ZENTRACLOUD_TOKEN")
, overwrite = TRUE
)
## force API call
system.time(
getReadings(
device_sn = "06-01185"
, token = getZentracloudToken()
, start_time = "2022-03-20 00:00:00"
, end_time = "2022-03-26 23:59:00"
, domain = "default"
, force = TRUE
)
)
#> [retrieving data]
#> [getting next page]
#> [retrieving data]
#> user system elapsed
#> 1.412 0.079 121.835
## get data from cache
system.time(
getReadings(
device_sn = "06-01185"
, token = getZentracloudToken()
, start_time = "2022-03-20 00:00:00"
, end_time = "2022-03-26 23:59:00"
, domain = "default"
, force = FALSE
)
)
#> user system elapsed
#> 0.453 0.016 59.457
More information
More detailed information on the package and all the functions can be found in the vignette:
vignette("zentracloud")